Class ResultsUserControl
- Namespace
- Loehnert.Lisrt.TypeAndResult.UserControls
- Assembly
- Loehnert.Lisrt.TypeAndResult.dll
Represents a control for displaying a collection of Results.
public class ResultsUserControl : UserControl, IAnimatable, IFrameworkInputElement, IInputElement, ISupportInitialize, IQueryAmbient, IAddChild
- Inheritance
-
ResultsUserControl
- Implements
- Inherited Members
Examples
Basic Usage
public class MyViewModel : PropertyChangedBase
{
public void SetMeasureCycle(MeasureCycle measureCycle)
{
// Show the results of all measure processes.
Results = measureCycle.MeasureProcesses.SelectMany(mp => mp.Results);
}
public IEnumerable<Result> Results { get; private set; }
}
Hide and Show Default Columns
<TypeAndResult:ResultsUserControl ItemsSource="{Binding Results}"
KeyColumnIsVisible="False"
GroupColumnIsVisible="True"/>
Customize Columns
To modify the columns, disable auto generating columns and add the columns by your self, for example using default column resources. You can also add custom columns, like the additional AttributeParameter column in the example below.
<TypeAndResult:ResultsUserControl ItemsSource="{Binding Results}"
AutoGenerateColumns="False">
<TypeAndResult:ResultsUserControl.Columns>
<!-- Add columns using default column resources -->
<StaticResource ResourceKey="ResultsDataGridValueColumn"/>
<StaticResource ResourceKey="ResultsDataGridKeyColumn"/>
<!-- Add a custom column -->
<DataGridTextColumn Header="Additional Attribute Parameter"
Binding="{Binding Attribute.Parameters[AttributeParameter].Text}"/>
</TypeAndResult:ResultsUserControl.Columns>
</TypeAndResult:ResultsUserControl>
Remarks
Resources for Default Columns
The package contains resources for default columns, use these to customize the Columns.
Key | Description |
---|---|
ResultsDataGridKeyColumn | Key of the attribute where the result depends on. |
ResultsDataGridGroupColumn | Group of the attribute where the result depends on. |
ResultsDataGridClassificationColumn | Classification of the result as image. |
ResultsDataGridLowerToleranceLimitColumn | Lower tolerance limit of the attribute where the result depends on. |
ResultsDataGridValueColumn | Value of the result. |
ResultsDataGridUpperToleranceLimitColumn | Upper tolerance limit of the attribute where the result depends on. |
ResultsDataGridUnitColumn | Unit of the attribute where the result depends on. |
ResultsDataGridTextColumn | Text of the result. |
ResultsDataGridTimeStampColumn | Time stamp of the result evaluation. |
ResultsDataGridGraphColumn | Result value, lower and upper limit as graphical representation. |
Constructors
ResultsUserControl()
Initializes a new instance of the ResultsUserControl class.
public ResultsUserControl()
Fields
AdditionalLimitColumnsAreVisibleProperty
Identifies the AdditionalLimitColumnsAreVisible dependency property.
public static readonly DependencyProperty AdditionalLimitColumnsAreVisibleProperty
Field Value
AutoGenerateColumnsProperty
Identifies the AutoGenerateColumns dependency property.
public static readonly DependencyProperty AutoGenerateColumnsProperty
Field Value
ClassificationColumnIsVisibleProperty
Identifies the ClassificationColumnIsVisible dependency property.
public static readonly DependencyProperty ClassificationColumnIsVisibleProperty
Field Value
FilterProperty
Identifies the Filter dependency property.
public static readonly DependencyProperty FilterProperty
Field Value
GraphColumnIsVisibleProperty
Identifies the GraphColumnIsVisible dependency property.
public static readonly DependencyProperty GraphColumnIsVisibleProperty
Field Value
GroupColumnIsVisibleProperty
Identifies the GroupColumnIsVisible dependency property.
public static readonly DependencyProperty GroupColumnIsVisibleProperty
Field Value
IsReadOnlyProperty
Identifies the IsReadOnly dependency property.
public static readonly DependencyProperty IsReadOnlyProperty
Field Value
ItemsSourceProperty
Identifies the ItemsSource dependency property.
public static readonly DependencyProperty ItemsSourceProperty
Field Value
KeyColumnIsVisibleProperty
Identifies the KeyColumnIsVisible dependency property.
public static readonly DependencyProperty KeyColumnIsVisibleProperty
Field Value
LowerToleranceLimitHeaderProperty
Identifies the LowerToleranceLimitHeaderProperty dependency property.
public static readonly DependencyProperty LowerToleranceLimitHeaderProperty
Field Value
ScrollToNewestRowProperty
Identifies the ScrollToNewestRow dependency property.
public static readonly DependencyProperty ScrollToNewestRowProperty
Field Value
TextColumnIsVisibleProperty
Identifies the TextColumnIsVisible dependency property.
public static readonly DependencyProperty TextColumnIsVisibleProperty
Field Value
TimeStampColumnIsVisibleProperty
Identifies the TimeStampColumnIsVisible dependency property.
public static readonly DependencyProperty TimeStampColumnIsVisibleProperty
Field Value
ToleranceColumnsAreVisibleProperty
Identifies the ToleranceColumnsAreVisible dependency property.
public static readonly DependencyProperty ToleranceColumnsAreVisibleProperty
Field Value
UnitColumnIsVisibleProperty
Identifies the UnitColumnIsVisible dependency property.
public static readonly DependencyProperty UnitColumnIsVisibleProperty
Field Value
UpperToleranceLimitHeaderProperty
Identifies the UpperToleranceLimitHeader dependency property.
public static readonly DependencyProperty UpperToleranceLimitHeaderProperty
Field Value
ValueColumnIsVisibleProperty
Identifies the ValueColumnIsVisible dependency property.
public static readonly DependencyProperty ValueColumnIsVisibleProperty
Field Value
Properties
AdditionalLimitColumnsAreVisible
Gets or sets a value indicating whether the AdditionalLimits of the Attribute are visible.
public bool AdditionalLimitColumnsAreVisible { get; set; }
Property Value
AutoGenerateColumns
Gets or sets a value indicating whether the columns are generated automatically. Setting to false, sets all ...ColumnIsVisible properties to true.
public bool AutoGenerateColumns { get; set; }
Property Value
ClassificationColumnIsVisible
Gets or sets a value indicating whether the Classification of the Result is visible.
public bool ClassificationColumnIsVisible { get; set; }
Property Value
Columns
Gets the columns.
public ObservableCollection<DataGridColumn> Columns { get; }
Property Value
Filter
Gets or sets a filter function.
public Predicate<object> Filter { get; set; }
Property Value
GraphColumnIsVisible
Gets or sets a value indicating whether the graph which shows the limits and the result value is visible.
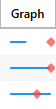
public bool GraphColumnIsVisible { get; set; }
Property Value
GroupColumnIsVisible
public bool GroupColumnIsVisible { get; set; }
Property Value
IsReadOnly
Gets or sets a value indicating whether the control is read only.
public bool IsReadOnly { get; set; }
Property Value
ItemsSource
Gets or sets the items source.
public IEnumerable<Result> ItemsSource { get; set; }
Property Value
KeyColumnIsVisible
public bool KeyColumnIsVisible { get; set; }
Property Value
LowerToleranceLimitHeader
Gets or sets the lower tolerance limit header.
public ITranslation LowerToleranceLimitHeader { get; set; }
Property Value
- ITranslation
ScrollToNewestRow
Gets or sets a value indicating whether to scroll to the newest row when adding a result.
public bool ScrollToNewestRow { get; set; }
Property Value
TextColumnIsVisible
public bool TextColumnIsVisible { get; set; }
Property Value
TimeStampColumnIsVisible
public bool TimeStampColumnIsVisible { get; set; }
Property Value
ToleranceColumnsAreVisible
public bool ToleranceColumnsAreVisible { get; set; }
Property Value
UnitColumnIsVisible
public bool UnitColumnIsVisible { get; set; }
Property Value
UpperToleranceLimitHeader
Gets or sets the upper tolerance limit header.
public ITranslation UpperToleranceLimitHeader { get; set; }
Property Value
- ITranslation
ValueColumnIsVisible
public bool ValueColumnIsVisible { get; set; }