Class MediaPlayer
Represents a media player to play video and audio files.
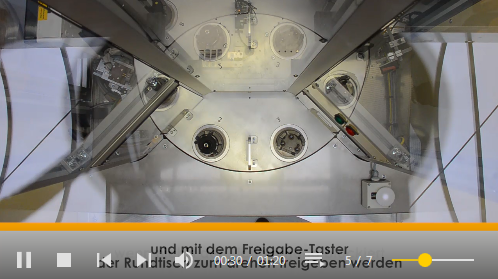
public class MediaPlayer : UserControl, IAnimatable, IFrameworkInputElement, IInputElement, ISupportInitialize, IQueryAmbient, IAddChild, INotifyPropertyChanged, IComponentConnector
- Inheritance
-
MediaPlayer
- Implements
- Inherited Members
Constructors
MediaPlayer()
Initializes a new instance of the MediaPlayer class.
public MediaPlayer()
Fields
AllowPlayPauseOnClickProperty
Identifies the AllowPlayPauseOnClick dependency property.
public static readonly DependencyProperty AllowPlayPauseOnClickProperty
Field Value
CanControlTimelineProperty
Identifies the CanControlTimeline dependency property.
public static readonly DependencyProperty CanControlTimelineProperty
Field Value
CurrentMediaIndexProperty
Identifies the CurrentMediaIndex dependency property.
public static readonly DependencyProperty CurrentMediaIndexProperty
Field Value
HasVideoProperty
Identifies the HasVideo dependency property.
public static readonly DependencyProperty HasVideoProperty
Field Value
IsAutoPlayProperty
Identifies the IsAutoPlay dependency property.
public static readonly DependencyProperty IsAutoPlayProperty
Field Value
IsMutedProperty
Identifies the IsMuted dependency property.
public static readonly DependencyProperty IsMutedProperty
Field Value
IsPausedProperty
Identifies the IsPaused dependency property.
public static readonly DependencyProperty IsPausedProperty
Field Value
IsPlayingProperty
Identifies the IsPlaying dependency property.
public static readonly DependencyProperty IsPlayingProperty
Field Value
IsStopedProperty
Identifies the IsStoped dependency property.
public static readonly DependencyProperty IsStopedProperty
Field Value
IsTransparentOnStopProperty
Identifies the IsTransparentOnStop dependency property.
public static readonly DependencyProperty IsTransparentOnStopProperty
Field Value
RepeatMediaProperty
Identifies the RepeatMedia dependency property.
public static readonly DependencyProperty RepeatMediaProperty
Field Value
RepeatQueueProperty
Identifies the RepeatQueue dependency property.
public static readonly DependencyProperty RepeatQueueProperty
Field Value
ShowControlBarProperty
Identifies the ShowControlBar dependency property.
public static readonly DependencyProperty ShowControlBarProperty
Field Value
ShowPlayPauseStateIconProperty
Identifies the ShowPlayPauseStateIcon dependency property.
public static readonly DependencyProperty ShowPlayPauseStateIconProperty
Field Value
ShowPlayProperty
Identifies the ShowPlay dependency property.
public static readonly DependencyProperty ShowPlayProperty
Field Value
ShowPlaylistProperty
Identifies the ShowPlaylist dependency property.
public static readonly DependencyProperty ShowPlaylistProperty
Field Value
ShowSkipControlsProperty
Identifies the ShowSkipControls dependency property.
public static readonly DependencyProperty ShowSkipControlsProperty
Field Value
ShowStopProperty
Identifies the ShowStop dependency property.
public static readonly DependencyProperty ShowStopProperty
Field Value
ShowTimeProperty
Identifies the ShowTime dependency property.
public static readonly DependencyProperty ShowTimeProperty
Field Value
ShowTimelineProperty
Identifies the ShowTimeline dependency property.
public static readonly DependencyProperty ShowTimelineProperty
Field Value
ShowVolumeProperty
Identifies the ShowVolume dependency property.
public static readonly DependencyProperty ShowVolumeProperty
Field Value
SourcesProperty
Identifies the Sources dependency property.
public static readonly DependencyProperty SourcesProperty
Field Value
VolumeProperty
Identifies the Volume dependency property.
public static readonly DependencyProperty VolumeProperty
Field Value
Properties
AllowPlayPauseOnClick
Gets or sets a value indicating whether play and pause on click/touch is enabled.
public bool AllowPlayPauseOnClick { get; set; }
Property Value
CanControlTimeline
Gets or sets a value indicating whether the timeline slider can be controlled.
public bool CanControlTimeline { get; set; }
Property Value
CurrentMediaIndex
Gets the index of current media (1 to TotalMedias).
public int CurrentMediaIndex { get; }
Property Value
HasVideo
Gets or sets a value indicating whether the media has video.
public bool HasVideo { get; set; }
Property Value
IsAutoPlay
Gets or sets a value indicating whether the media starts playing on load.
public bool IsAutoPlay { get; set; }
Property Value
IsMuted
Gets or sets a value indicating whether the audio is muted.
public bool IsMuted { get; set; }
Property Value
IsPaused
Gets or sets a value indicating whether the media is paused.
public bool IsPaused { get; set; }
Property Value
IsPlaying
Gets or sets a value indicating whether the media is playing.
public bool IsPlaying { get; set; }
Property Value
IsStoped
Gets or sets a value indicating whether the media is stopped.
public bool IsStoped { get; set; }
Property Value
IsTransparentOnStop
Gets or sets a value indicating whether the video is transparent on stop (not the controls).
public bool IsTransparentOnStop { get; set; }
Property Value
PlaylistItems
Gets the play list items.
public IEnumerable<string> PlaylistItems { get; }
Property Value
RepeatMedia
Gets or sets a value indicating whether the media is repeated at the end.
public bool RepeatMedia { get; set; }
Property Value
RepeatQueue
Gets or sets a value indicating whether the playlist is repeated.
public bool RepeatQueue { get; set; }
Property Value
ShowControlBar
Gets or sets a value indicating whether the control bar is shown.
public bool ShowControlBar { get; set; }
Property Value
ShowPlay
Gets or sets a value indicating whether the play and stop button is shown.
public bool ShowPlay { get; set; }
Property Value
ShowPlayPauseStateIcon
Gets or sets a value indicating whether the play and pause state icon is shown.
public bool ShowPlayPauseStateIcon { get; set; }
Property Value
ShowPlaylist
Gets or sets a value indicating whether the playlist icon is shown.
public bool ShowPlaylist { get; set; }
Property Value
ShowSkipControls
Gets or sets a value indicating whether the skip controls are shown.
public bool ShowSkipControls { get; set; }
Property Value
ShowStop
Gets or sets a value indicating whether the stop button is shown.
public bool ShowStop { get; set; }
Property Value
ShowTime
Gets or sets a value indicating whether the time control is shown.
public bool ShowTime { get; set; }
Property Value
ShowTimeline
Gets or sets a value indicating whether the timeline control is shown.
public bool ShowTimeline { get; set; }
Property Value
ShowVolume
Gets or sets a value indicating whether the volume control is shown.
public bool ShowVolume { get; set; }
Property Value
Source
Gets the current media URI.
public Uri Source { get; }
Property Value
Sources
Gets or sets the uris of the media sources. Do not use embedded resources.
public IEnumerable<Uri> Sources { get; set; }
Property Value
SpeedRatio
Gets or sets the speed ratio of the media. The speed ratio of the media. The valid range is between 0 (zero) and infinity. Values less than 1 yield slower than normal playback, and values greater than 1 yield faster than normal playback. Negative values are treated as 0. The default value is 1.
public double SpeedRatio { get; set; }
Property Value
TotalMedias
Gets the numbers of all medias in queue.
public int TotalMedias { get; }
Property Value
Volume
Gets or sets the media's volume (0 to 1).
public double Volume { get; set; }
Property Value
Methods
InitializeComponent()
InitializeComponent
public void InitializeComponent()
JumpToNext()
Jump to next media.
public void JumpToNext()
JumpToPrevious()
Jump to previous media.
public void JumpToPrevious()
Pause()
Pauses media at the current position.
public void Pause()
Play()
Plays media from the current position.
public void Play()
Stop()
Stops and resets media to be played from the beginning.
public void Stop()
Events
PropertyChanged
Occurs when a property value changes.
public event PropertyChangedEventHandler PropertyChanged